MongoDB - Auto Increment Sequence
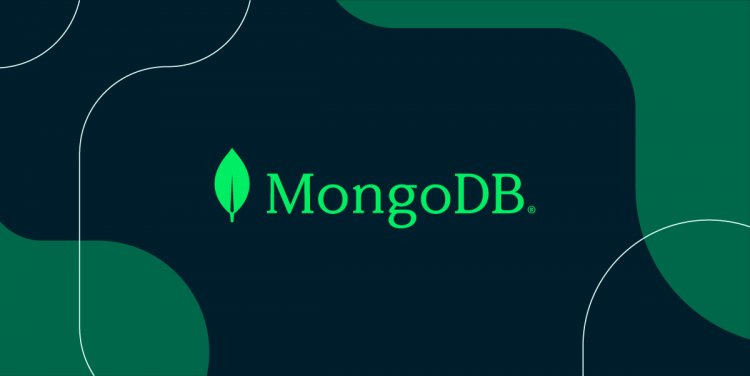
MongoDB - Auto Increment Sequence
When it comes to databases, MongoDB is a popular choice due to its flexibility, scalability, and document-oriented structure. However, if you're accustomed to SQL databases, you might have noticed that MongoDB lacks out-of-the-box auto-increment functionality for primary keys. In MongoDB, the default primary key is a 12-byte ObjectId used to uniquely identify documents. But fear not, there's a way to achieve auto-incremented values for fields like _id
. In this article, we'll explore how to programmatically implement auto-increment functionality in MongoDB using a counters collection, as recommended by the MongoDB documentation.
Understanding the Challenge
In SQL databases, auto-incrementing fields are a breeze. You simply define a column as AUTO_INCREMENT
, and the database takes care of the rest. MongoDB, however, uses a different approach with its ObjectId primary keys. While these ObjectIds are unique and perform well in distributed environments, there are cases where you might prefer a more traditional auto-incremented value for your _id
field.
The Solution: Counters Collection
To overcome this limitation, MongoDB suggests using a counters collection. This collection will store a document that holds the current value of the auto-incremented field. Here's a step-by-step guide on how to implement this solution:
1. Create a Counters Collection
Start by creating a counters collection in your MongoDB database. You can do this using the MongoDB shell or a MongoDB client library in your programming language of choice.
db.createCollection("counters")
2. Initialize the Counter
Insert an initial document in the counters collection with a field for the auto-incremented value. This document will serve as the starting point for your auto-incremented field.
db.counters.insertOne({ _id: "productId", // Use a unique name for your auto-incremented field sequence_value: 0 // Initial value })
3. Create a Function
Next, create a function in your application code to fetch and increment the counter value whenever you need to insert a new document. Here's a JavaScript example using the Node.js MongoDB driver:
function getNextSequenceValue(sequenceName)
{
var sequenceDocument = db.counters.findAndModify( { query:{_id: sequenceName }, update: {$inc:{sequence_value:1}}, new:true });
return sequenceDocument.sequence_value;
}
4. Implement Auto-Increment
Now, whenever you want to insert a new document with an auto-incremented _id
, you can call the getNextSequenceValue
function to get the next value:
var nextProductId = getNextSequenceValue("productId");
db.products.insert({ _id: nextProductId, name: "Product Name", // Other fields })
Benefits of Using Counters
Implementing auto-increment with a counters collection provides several advantages:
- Control: You have full control over the auto-increment process, including initialization and naming conventions.
- Scalability: This approach is scalable and performs well in distributed environments.
- Compatibility: It allows you to maintain compatibility with existing systems that rely on auto-incremented primary keys.
Conclusion
While MongoDB doesn't offer out-of-the-box auto-increment functionality, it provides a robust alternative through the use of counters collections. By following the steps outlined in this article, you can easily implement auto-incremented fields in your MongoDB documents, giving you the best of both worlds: the power of MongoDB and the familiarity of auto-incremented values.
FAQs
-
Is it possible to use auto-increment with other fields, not just
_id
?- Yes, you can implement auto-increment for any field in your documents by following a similar approach with separate counters collections.
-
Do I need to manually manage the counter values in a production environment?
- It's recommended to handle counter management within your application code to ensure data integrity and consistency.
-
Can I use auto-incremented values for existing documents?
- While you can implement auto-increment for new documents, it may require some data migration efforts to apply it to existing documents.
-
Are there any performance considerations when using counters collections?
- MongoDB's performance with counters collections is generally efficient, but it's essential to monitor and optimize your implementation for high traffic scenarios.
-
Is there an official MongoDB package or library for handling auto-increment?
- MongoDB does not provide an official auto-increment package, as it encourages developers to implement this feature programmatically to suit their specific use cases.