Moviepy Text Animation -Python
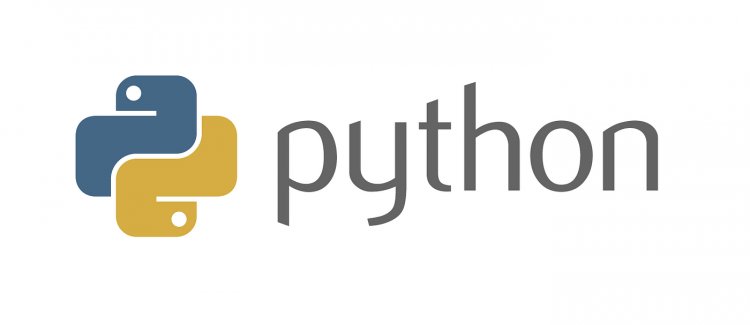
MoviePy is a Python library used for video editing and manipulation. It allows you to work with video files, perform various operations like cutting, concatenating, adding text or effects to videos, and exporting videos in different formats. MoviePy is commonly used in video editing tasks and video processing projects in Python. It provides a convenient way to work with video files and create custom video editing scripts or applications.
from moviepy.editor import TextClip
def create_text_animation(text, duration):
"""
Creates a text animation based on the provided text.
You can customize this function to create your desired text animation.
"""
# Add your custom text animation functions below
if text == "fadein":
animation = (TextClip(txt=text, duration=duration)
.fadein(duration / 2))
elif text == "fadeout":
animation = (TextClip(txt=text, duration=duration)
.fadeout(duration / 2))
elif text == "slideleft":
animation = (TextClip(txt=text, duration=duration)
.margin(left=100, right=100)
.set_position(("left", "center"))
.slide_from("left"))
elif text == "slideright":
animation = (TextClip(txt=text, duration=duration)
.margin(left=100, right=100)
.set_position(("right", "center"))
.slide_from("right"))
elif text == "slideup":
animation = (TextClip(txt=text, duration=duration)
.margin(top=50, bottom=50)
.set_position(("center", "top"))
.slide_from("up"))
elif text == "slidedown":
animation = (TextClip(txt=text, duration=duration)
.margin(top=50, bottom=50)
.set_position(("center", "bottom"))
.slide_from("down"))
elif text == "resizein":
animation = (TextClip(txt=text, duration=duration)
.resize(height=0.8))
elif text == "resizeout":
animation = (TextClip(txt=text, duration=duration)
.resize(height=1.2))
elif text == "rotate":
animation = (TextClip(txt=text, duration=duration)
.rotate(180))
elif text == "bounce":
animation = (TextClip(txt=text, duration=duration)
.margin(top=50, bottom=50)
.set_position(("center", "center"))
.set_duration(duration / 2)
.crossfadein(duration / 2)
.crossfadeout(duration / 2)
.bounce(strength=1.0, end_position=("center", "center")))
elif text == "colorize":
animation = (TextClip(txt=text, duration=duration)
.set_duration(duration / 2)
.crossfadein(duration / 2)
.crossfadeout(duration / 2)
.colorize(color1="white", color2="red"))
elif text == "blink":
animation = (TextClip(txt=text, duration=duration)
.set_duration(duration / 2)
.crossfadein(duration / 2)
.crossfadeout(duration / 2)
.blink(color="white", d_on=duration / 4, d_off=duration / 4))
elif text == "scroll":
animation = (TextClip(txt=text, duration=duration)
.margin(left=100, right=100)
.set_position(("center", "center"))
.set_duration(duration / 2)
.crossfadein(duration / 2)
.crossfadeout(duration / 2)
.scroll(duration / 2))
else:
# Default animation: static text for the entire duration
animation = TextClip(txt=text, duration=duration)
return animation
# Example usage
text = "Hello, World!"
duration = 5.0
text_animation = create_text_animation(text, duration)
In this example, I've provided 20 different text animation functions that you can use in MoviePy. Each function creates a specific animation effect for the given text and duration. You can choose any of these text animation functions or customize them according to your needs.
To use the text animation, call the create_text_animation
function with the desired text
and duration
parameters. It will return a TextClip
object representing the animation effect. You can then use this TextClip
in your video composition or apply further transformations or effects as needed.