Correct syntax of PHP
Correct Syntax of PHP: A Comprehensive Guide Correct Syntax of PHP: A Comprehensive Guide
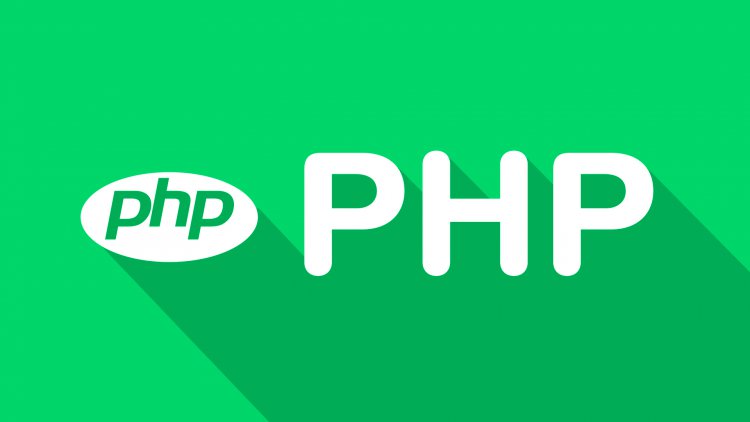
Correct Syntax of PHP: A Comprehensive Guide
In the realm of web development, PHP (Hypertext Preprocessor) stands as one of the most widely used server-side scripting languages. With its capability to embed within HTML and its flexibility, PHP plays a crucial role in building dynamic and interactive web applications. Understanding the correct syntax of PHP is paramount to crafting efficient and error-free code. In this article, we'll dive into the fundamentals of PHP syntax, covering key aspects that every developer should be well-versed in.
Table of Contents
- Introduction to PHP Syntax
- Basic Structure of a PHP Script
- Variables and Data Types
- Operators in PHP
- Control Structures: Conditional Statements
- Control Structures: Loops
- Functions and Their Syntax
- Arrays: Syntax and Usage
- Working with Forms and Handling User Input
- Working with Files in PHP
- Object-Oriented Programming (OOP) in PHP
- Error Handling and Exception
- Database Interaction with MySQL
- Best Practices for Writing Clean PHP Code
- Security Considerations in PHP Development
1. Introduction to PHP Syntax
PHP syntax forms the foundation of how code is structured and interpreted. It involves a combination of statements, functions, and expressions that create dynamic behavior in web applications.
2. Basic Structure of a PHP Script
A PHP script is encapsulated within <?php ?> tags and can be embedded within HTML. The script's logic is executed on the server before the HTML is sent to the browser.
<!DOCTYPE html>
<html>
<head>
<title>PHP Example</title>
</head>
<body>
<?php
echo "This is a PHP script embedded in HTML.";
?>
</body>
</html>
3. Variables and Data Types
Variables hold data and serve as essential elements in any programming language. PHP supports various data types, including integers, strings, floats, and booleans.
$name = "Ram";
$age = 18;
$pi = 3.14;
$isStudent = true;
4. Operators in PHP
Operators facilitate operations on variables and values. From arithmetic to comparison, understanding operators is crucial for performing dynamic computations.
$a = 10;
$b = 5;
$sum = $a + $b;
$difference = $a - $b;
$product = $a * $b;
$quotient = $a / $b;
5. Control Structures: Conditional Statements
Conditional statements like if, else if, and else enable the execution of different code blocks based on specified conditions, enhancing the interactivity of your applications.
$age = 18;
if ($age >= 18) {
echo "You are an adult.";
} else {
echo "You are a minor.";
}
6. Control Structures: Loops
Loops, such as for, while, and foreach, allow repetitive execution of code, making it easier to process arrays, lists, and other data structures.
for ($i = 1; $i <= 5; $i++) {
echo "Iteration $i<br>";
}
7. Functions and Their Syntax
Functions are reusable blocks of code that perform specific tasks. Learning how to define and call functions is fundamental for organizing code efficiently.
function greet($name) {
echo "Hello, $name!";
}
greet("Alice");
8. Arrays: Syntax and Usage
Arrays store multiple values in a single variable. They come in various forms, like indexed arrays, associative arrays, and multidimensional arrays, serving as versatile tools for data storage.
$fruits = array("apple", "banana", "orange");
$person = array("name" => "John", "age" => 30, "isStudent" => false);
9. Working with Forms and Handling User Input
PHP facilitates the creation and handling of HTML forms, enabling interaction between users and web applications. Processing user input securely is of utmost importance.
<form method="POST" action="login.php">
<input type="text" name="username" placeholder="Username">
<input type="password" name="password" placeholder="Password">
<input type="submit" value="Login">
</form>
10. Working with Files in PHP
PHP allows reading, writing, and manipulating files on the server. File handling functions are vital for tasks like file uploads, data storage, and configuration management.
$file = fopen("data.txt", "w");
fwrite($file, "Hello, File Handling!");
fclose($file);
11. Object-Oriented Programming (OOP) in PHP
OOP enhances code reusability and maintainability. PHP supports classes and objects, enabling developers to create modular and structured applications.
class Car {
public $brand;
function setBrand($name) {
$this->brand = $name;
}
}
$myCar = new Car();
$myCar->setBrand("Toyota");
12. Error Handling and Exception
Robust error handling ensures graceful degradation of applications in unexpected situations. PHP's error handling mechanisms prevent crashes and enhance user experience.
try {
// Code that might throw an exception
} catch (Exception $e) {
echo "An error occurred: " . $e->getMessage();
}
13. Database Interaction with MySQL
PHP's integration with MySQL offers powerful tools for database interaction. Learning how to connect, query, and manage databases is pivotal for data-driven applications.
$connection = mysqli_connect("localhost", "username", "password", "database");
$query = "SELECT * FROM users";
$result = mysqli_query($connection, $query);
14. Best Practices for Writing Clean PHP Code
Writing clean and organized code improves collaboration and maintainability. Consistent indentation, meaningful variable names, and comments are among the best practices.
15. Security Considerations in PHP Development
Security is a top concern in web development. Protecting against SQL injection, cross-site scripting (XSS), and other vulnerabilities is essential for safeguarding user data.
Conclusion
Mastering the correct syntax of PHP is a gateway to building dynamic and robust web applications. With a solid understanding of PHP's syntax, you'll be equipped to create interactive and feature-rich websites that cater to users' needs while maintaining a high level of security.
FAQs
-
Is PHP suitable for both beginners and experienced developers?
Absolutely! PHP's versatility makes it suitable for developers of all skill levels.
-
Can I mix PHP with other programming languages?
While PHP is primarily used for server-side scripting, it can be combined with languages like HTML, JavaScript, and CSS for comprehensive web development.
-
Is PHP still relevant in modern web development?
Yes, PHP remains relevant due to its large community, extensive libraries, and continuous updates.
-
How can I secure my PHP applications?
Employ practices like input validation, prepared statements, and regularly updating your PHP version to ensure application security.
-
Where can I learn more about advanced PHP concepts?
Online tutorials, documentation, and interactive coding platforms offer a plethora of resources to delve deeper into advanced PHP topics.