Power Detection in Firmware: A Microcontroller Implementation Guide
Learn how to implement power detection in firmware using a microcontroller. Utilize hardware interfaces and programming languages specific to your microcontroller platform. Explore a comprehensive example in C language for Arduino boards, leveraging the ADC (Analog-to-Digital Converter) module for monitoring power levels. Master the process of detecting power in your firmware with step-by-step instructions and practical code examples.
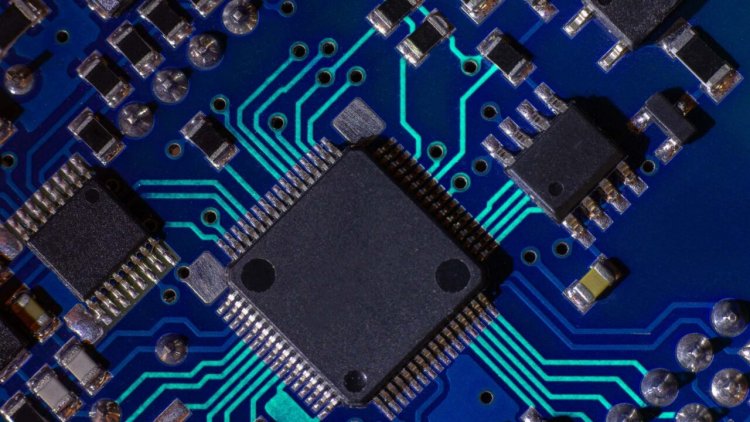
Power Detection in Firmware: A Microcontroller Implementation Guide
Introduction
In the world of embedded systems, efficient power management is essential. Microcontrollers play a pivotal role in this, as they often control devices that need to conserve power to extend battery life or operate in energy-sensitive environments. This article dives deep into power detection in firmware, providing you with a comprehensive guide on implementing this critical feature. We'll explore how to detect power states, optimize power consumption, and ensure your microcontroller operates efficiently.
Understanding Power Modes
Power modes are a cornerstone of microcontroller design. They determine how the device consumes energy and can significantly impact battery life. Let's delve into different power modes:
Hibernation Mode
Hibernation mode is the lowest power state, where the microcontroller nearly shuts down but retains essential data. Learn how to implement and exit hibernation mode.
Sleep Mode
Discover the nuances of sleep mode, which conserves power while keeping some peripherals active for rapid wake-up.
Voltage and Current Monitoring
Monitoring voltage and current is crucial for efficient power management. Let's explore the tools and techniques available:
ADC (Analog-to-Digital Converter) Implementation
Learn how to use ADC to monitor voltage levels and make informed decisions based on the readings.
Current Sensing Techniques
Discover various current sensing methods, such as shunt resistors and Hall-effect sensors, to monitor power consumption.
Implementing Low-Power Algorithms
Optimizing firmware is essential for power efficiency. We'll discuss several strategies:
Idle Loops
Explore how idle loops can save power by minimizing CPU activity during idle states.
Dynamic Voltage Scaling
Learn about dynamic voltage scaling to adapt the microcontroller's operating voltage to the current workload.
Wake-up Sources
Understanding wake-up sources is crucial for responsive, power-efficient systems:
External Interrupts
Discover how external events can trigger the microcontroller to wake up from low-power states.
Timers and Watchdogs
Learn to utilize timers and watchdogs for scheduled wake-ups and system monitoring.
Power-Down Modes
Power-down modes are an effective way to reduce power consumption during inactivity:
Standby Mode
Implement standby mode to reduce power consumption while still maintaining quick response times.
Shutdown Mode
Explore shutdown mode, where the microcontroller shuts down all functions except for external wake-up sources.
To implement power detection in firmware using a microcontroller, you can utilize the appropriate hardware interfaces and programming language specific to your microcontroller platform. Here's a general example in C language for an Arduino board using the ADC (Analog-to-Digital Converter) module to monitor the power level:
// Pin connected to power monitoring circuit
#define POWER_MONITOR_PIN A0
// Threshold for power detection (adjust as needed)
#define POWER_THRESHOLD 500
void setup() {
// Initialize the ADC
analogReadResolution(10);
analogReference(DEFAULT);
// Initialize the serial communication
Serial.begin(9600);
}
void loop() {
// Read the analog value from the power monitor pin
int powerLevel = analogRead(POWER_MONITOR_PIN);
// Check if power level exceeds the threshold
if (powerLevel > POWER_THRESHOLD) {
// Power detected
Serial.println("Power detected!");
// Perform necessary actions or trigger an event
}
// Delay between readings
delay(1000);
}
In this example, the code monitors the power level by reading the analog value from the specified pin (A0
in this case) connected to the power monitoring circuit. The power level is compared against a predefined threshold (POWER_THRESHOLD
), which you can adjust based on your requirements.
Here's a breakdown of the code:
-
The
setup()
function initializes the ADC by setting the resolution and reference voltage. It also initializes the serial communication for debugging or reporting purposes. -
The
loop()
function is the main execution loop that repeatedly reads the analog value from the power monitor pin usinganalogRead()
. The obtained value is stored in thepowerLevel
variable. -
The
powerLevel
value is then compared against thePOWER_THRESHOLD
. If the power level exceeds the threshold, a message is printed to the serial monitor indicating the detection of power. You can replace the serial output with the desired actions or events triggered upon power detection. -
A delay of 1 second (
delay(1000)
) is added between readings to control the sampling rate. You can adjust the delay duration according to your needs.
Please note that the above example is a general template and may require modification based on the specific microcontroller platform and firmware development environment you are using. The pin numbers, ADC settings, and threshold values might differ, so make sure to refer to the appropriate documentation and adapt the code to your specific hardware and programming language.
Conclusion
Efficient power management in microcontroller firmware is critical for extending battery life and improving system responsiveness. By understanding power modes, monitoring voltage and current, implementing low-power algorithms, and using appropriate wake-up sources and power-down modes, you can create energy-efficient devices that excel in various applications.
FAQs
-
What is the primary benefit of hibernation mode in microcontroller firmware?
- Hibernation mode allows the microcontroller to consume minimal power while retaining critical data, making it ideal for applications with stringent power constraints.
-
How does dynamic voltage scaling impact power consumption?
- Dynamic voltage scaling adjusts the microcontroller's operating voltage to match the workload, reducing power consumption during periods of low activity.
-
What is the difference between standby mode and shutdown mode?
- Standby mode reduces power consumption while maintaining quick response times, while shutdown mode shuts down all functions except for external wake-up sources, consuming the least amount of power.
-
Can I use external interrupts to wake up a microcontroller from sleep mode?
- Yes, external interrupts can trigger the microcontroller to wake up from sleep mode, allowing for responsive operation.
-
What are some common current sensing techniques used in microcontroller applications?
- Common current sensing techniques include shunt resistors and Hall-effect sensors, which help monitor power consumption accurately.
Now armed with this knowledge, you can effectively implement power detection in your microcontroller firmware, ensuring your devices operate efficiently and conservatively in terms of power consumption.