Working with Regular Expressions in PHP
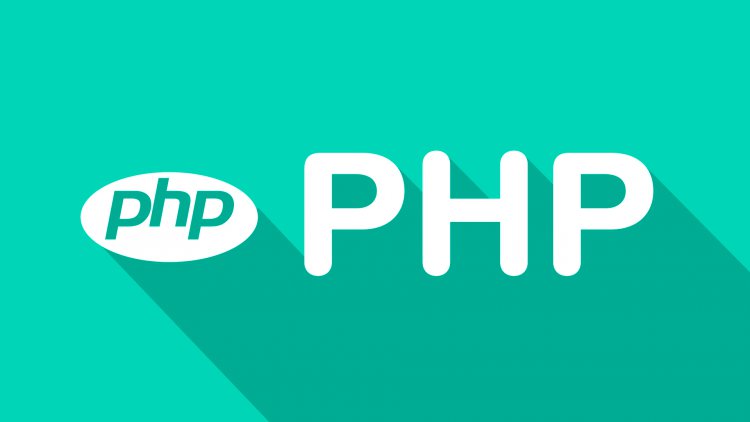
Introduction
In this comprehensive guide, we will delve into the intricacies of working with regular expressions in PHP. Regular expressions, often referred to as regex, are powerful tools for pattern matching and text manipulation. Understanding how to utilize them effectively can significantly enhance your programming skills and allow you to solve complex text processing problems efficiently.
What Are Regular Expressions?
Regular expressions are sequences of characters that define a search pattern. They are primarily used to search, replace, and validate strings based on specific rules or patterns. Regular expressions are widely supported in various programming languages, including PHP, and provide a flexible and efficient way to perform pattern matching operations.
Benefits of Using Regular Expressions
Regular expressions offer numerous benefits when working with text processing tasks in PHP:
- Pattern Matching: Regular expressions enable you to search for patterns within strings, making it easier to extract and manipulate specific data.
- String Validation: You can validate user input against predefined patterns, ensuring that the data meets specific criteria.
- Text Manipulation: By using regular expressions, you can easily replace or modify specific parts of a string, saving time and effort.
- Flexible Searching: Regular expressions provide powerful search capabilities, allowing you to find complex patterns or combinations of characters efficiently.
Understanding Regular Expression Syntax
To effectively work with regular expressions in PHP, it is crucial to understand their syntax. Regular expressions consist of a combination of characters and metacharacters that define the pattern to match.
Here are some essential components of regular expressions:
- Literal Characters: These are characters that match themselves. For example, the regular expression
/hello/
matches the string "hello" exactly. - Metacharacters: Metacharacters have special meanings in regular expressions. They allow you to define more complex patterns. Some commonly used metacharacters in PHP include
.
(dot),*
(asterisk),+
(plus),?
(question mark),[]
(character class), and()
(grouping). - Quantifiers: Quantifiers specify the number of occurrences a pattern should match. They include
*
(zero or more occurrences),+
(one or more occurrences),?
(zero or one occurrence), and{}
(exact number of occurrences). - Anchors: Anchors are used to match patterns at specific positions within a string. The most common anchors are
^
(start of the string) and$
(end of the string).
Working with Regular Expressions in PHP
To utilize regular expressions in PHP, we can use the built-in functions and methods provided by the preg
family of functions. Here are some commonly used functions for working with regular expressions:
- preg_match(): This function allows you to perform a pattern match against a string and returns a boolean value indicating whether the pattern was found or not.
- preg_match_all(): Similar to
preg_match()
, this function matches all occurrences of a pattern within a string and returns the number of matches. - preg_replace(): This function replaces all occurrences of a pattern in a string with a specified replacement value.
- preg_split(): Using this function, you can split a string into an array based on a specified pattern.
Example: Matching Email Addresses
Let's illustrate the practical use of regular expressions in PHP by matching email addresses within a string. Consider the following example:
$text = "Contact us at support@example.com or info@example.com for assistance.";
$pattern = "/\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,}\b/";
preg_match_all($pattern, $text, $matches);
print_r($matches[0]);
preg_match_all()
function searches the $text
string for all occurrences of the pattern and stores the matches in the $matches
array. Finally, we print the matched email addresses.Example: Replacing HTML Tags
Another practical application of regular expressions is replacing HTML tags within a string. Consider the following example:
$html = "
Hello, world!
";
$pattern = "/<[^>]+>/";
$cleanText = preg_replace($pattern, "", $html);
echo $cleanText;
In this example, we define a pattern that matches any HTML tags. The preg_replace()
function removes all HTML tags from the $html
string, resulting in clean text. Finally, we echo the cleaned text.
RegExp Generator
Generated RegExp:
Conclusion
In this comprehensive guide, we explored the power and versatility of working with regular expressions in PHP. By understanding the syntax and utilizing the various functions available, you can unlock the full potential of regular expressions and tackle complex text processing tasks effectively. Regular expressions are a valuable tool in any PHP developer's arsenal, enabling efficient pattern matching, string validation, and text manipulation.
Remember, mastering regular expressions takes practice and experience. Continuously exploring and experimenting with different patterns and use cases will further enhance your skills in working with regular expressions in PHP.