Working with MySQL in PHP
connection with mysql using php, php mysql extension , php and mysql notes Working with MySQL in PHP: A Comprehensive Guide
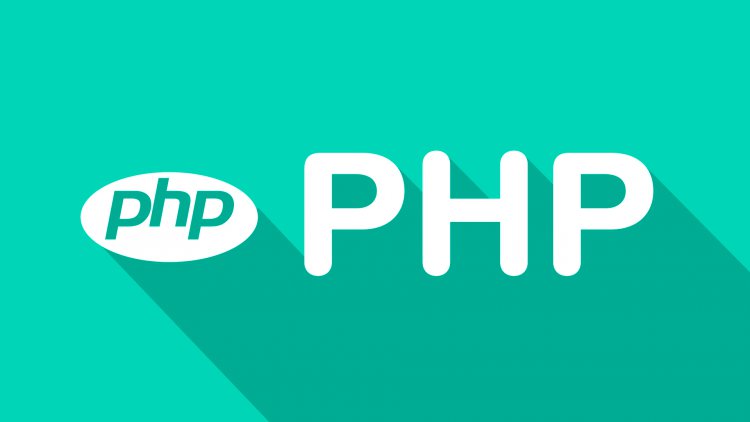
Working with MySQL in PHP: A Comprehensive Guide
In the world of web development, databases play a pivotal role in storing and managing data. MySQL, a popular open-source relational database management system, is widely used for its efficiency and scalability. Integrating MySQL with PHP allows developers to create dynamic and data-driven web applications. In this article, we will delve into the process of working with MySQL in PHP, covering essential concepts and techniques.
Table of Contents
- Introduction to MySQL and PHP Integration
- Setting Up a MySQL Database
- Establishing Database Connection in PHP
- Executing SQL Queries
- Retrieving Data from the Database
- Inserting and Updating Records
- Prepared Statements for Security
- Managing Transactions
- Working with Joins for Complex Queries
- Handling Errors and Exceptions
- Best Practices for MySQL and PHP Development
- Optimizing Database Performance
- Security Considerations
- Scaling MySQL and PHP Applications
- Conclusion
1. Introduction to MySQL and PHP Integration
MySQL and PHP are a powerful duo for building web applications that require efficient data storage and retrieval. PHP's ability to dynamically generate SQL queries makes it an ideal companion for MySQL.
2. Setting Up a MySQL Database
To start, you need to create a MySQL database. You can use tools like phpMyAdmin or command-line interfaces to set up your database structure.
Example:
CREATE DATABASE mydatabase;
3. Establishing Database Connection in PHP
Connecting to a MySQL database in PHP requires using functions like mysqli_connect()
or PDO (PHP Data Objects) to establish a connection.
Example using mysqli:
$hostname = "localhost"; $username = "root"; $password = "password"; $database = "mydatabase";
$connection = mysqli_connect($hostname, $username, $password, $database);
if (!$connection) {
die("Connection failed: " . mysqli_connect_error());
}
4. Executing SQL Queries
PHP allows you to execute SQL queries using functions like mysqli_query()
or PDO's query()
. This enables you to perform operations like creating tables, altering schemas, and more.
Example using mysqli:
$query = "CREATE TABLE users ( id INT AUTO_INCREMENT PRIMARY KEY, username VARCHAR(50) NOT NULL, email VARCHAR(100) NOT NULL )";
if (mysqli_query($connection, $query)) {
echo "Table created successfully.";
} else {
echo "Error creating table: " . mysqli_error($connection);
}
5. Retrieving Data from the Database
Fetching data from the database involves executing SELECT queries and then iterating through the result set to extract the desired information.
Example using mysqli:
$query = "SELECT * FROM users"; $result = mysqli_query($connection, $query);
if (mysqli_num_rows($result) > 0) {
while ($row = mysqli_fetch_assoc($result)) {
echo "ID: " . $row["id"] . " - Username: " . $row["username"] . " - Email: " . $row["email"] . "
";
}
} else {
echo "No results found.";
}
6. Inserting and Updating Records
You can insert new records using INSERT queries and update existing records using UPDATE queries. This is essential for managing user data and content.
Example using mysqli for insertion:
$insertQuery = "INSERT INTO users (username, email) VALUES ('john_doe', 'john@example.com')";
if (mysqli_query($connection, $insertQuery)) {
echo "Record inserted successfully.";
} else {
echo "Error inserting record: " . mysqli_error($connection);
}
Example using mysqli for updating:
$updateQuery = "UPDATE users SET email='new_email@example.com' WHERE id=1";
if (mysqli_query($connection, $updateQuery)) {
echo "Record updated successfully.";
} else {
echo "Error updating record: " . mysqli_error($connection);
}
7. Prepared Statements for Security
To prevent SQL injection attacks, use prepared statements with placeholders. This binds parameters securely before executing queries.
Example using prepared statements:
$preparedQuery = "INSERT INTO users (username, email) VALUES (?, ?)";
$stmt = mysqli_prepare($connection, $preparedQuery);
mysqli_stmt_bind_param($stmt, "ss", $username, $email);
$username = "alice"; $email = "alice@example.com";
if (mysqli_stmt_execute($stmt)) {
echo "Record inserted using prepared statement.";
} else {
echo "Error inserting record: " . mysqli_error($connection);
}
mysqli_stmt_close($stmt);
8. Managing Transactions
Transactions ensure data integrity. Begin a transaction, perform multiple operations, and commit the changes, or roll them back if an error occurs.
Example using transactions:
mysqli_autocommit($connection, false);
$insertQuery = "INSERT INTO users (username, email) VALUES ('bob', 'bob@example.com')";
$updateQuery = "UPDATE users SET email='updated_email@example.com' WHERE id=3";
if (mysqli_query($connection, $insertQuery) && mysqli_query($connection, $updateQuery))
{
mysqli_commit($connection); echo "Transaction successful.";
} else {
mysqli_rollback($connection);
echo "Transaction rolled back.";
}
mysqli_autocommit($connection, true);
9. Working with Joins for Complex Queries
Joins allow you to combine data from multiple tables, facilitating complex queries that retrieve specific information from related tables.
Example using JOIN:
SELECT users.username, orders.order_id, orders.total_amount FROM users INNER JOIN orders ON users.id = orders.user_id;
10. Handling Errors and Exceptions
Effective error handling enhances application reliability. Utilize try-catch blocks to capture exceptions and provide meaningful error messages.
Example using try-catch:
try {
// Code that might throw an exception
} catch (Exception $e) {
echo "An error occurred: " . $e->getMessage();
}
11. Best Practices for MySQL and PHP Development
Adhere to best practices such as organizing code, using meaningful variable names, and commenting to enhance code readability and maintainability.
12. Optimizing Database Performance
Efficient database design, indexing, and query optimization contribute to improved performance and faster response times.
13. Security Considerations
Implement security measures like input validation, escaping user inputs, and limiting database privileges to safeguard sensitive data.
14. Scaling MySQL and PHP Applications
As your application grows, consider strategies like load balancing, caching, and database sharding to handle increased traffic and data.
Conclusion
Working with MySQL in PHP opens up a world of possibilities for dynamic and data-driven web applications. The integration of these technologies empowers developers to create feature-rich platforms that efficiently manage data while providing a seamless user experience.
FAQs
-
Is MySQL the only option for PHP database integration?
No, other databases like PostgreSQL and SQLite can also be integrated with PHP.
-
What is the advantage of using prepared statements?
Prepared statements prevent SQL injection by separating user input from the query itself.
-
Can I use MySQL for non-PHP applications?
Yes, MySQL is versatile and can be used with a variety of programming languages and applications.
-
How can I improve the performance of my MySQL database?
Optimize queries, use appropriate indexing, and consider implementing caching mechanisms.
-
Is MySQL suitable for large-scale applications?
Yes, MySQL's scalability features and efficient handling of data make it suitable for both small and large applications.