check an IP address is within a range in PHP?
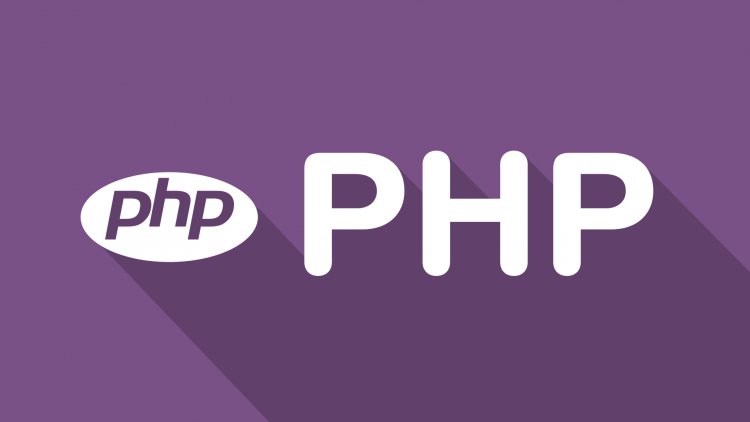
In this article i am going to explain you " To check an IP address is within a range in PHP?". for this you must have to use a IP range eg:127.0.0.1/24. this structure called IP range.
IP / Range
/**
* Checks if a given IP address matches the specified CIDR subnet/s
*
* @param string $ip The IP address to check
* @param mixed $cidrs The IP subnet (string) or subnets (array) in CIDR notation
* @param string $match optional If provided, will contain the first matched IP subnet
* @return boolean TRUE if the IP matches a given subnet or FALSE if it does not
*/
With ip2long() it's easy to convert your addresses to numbers. After this, you just have to check if the number is in range:
if ($ip <= $high_ip && $low_ip <= $ip) { echo "in range"; }Hope this article "To check an IP address is within a range in PHP?" will help for you. please comment and share with your friends.