AES Encryption and Decryption in PHP
AES Encryption and Decryption in PHP
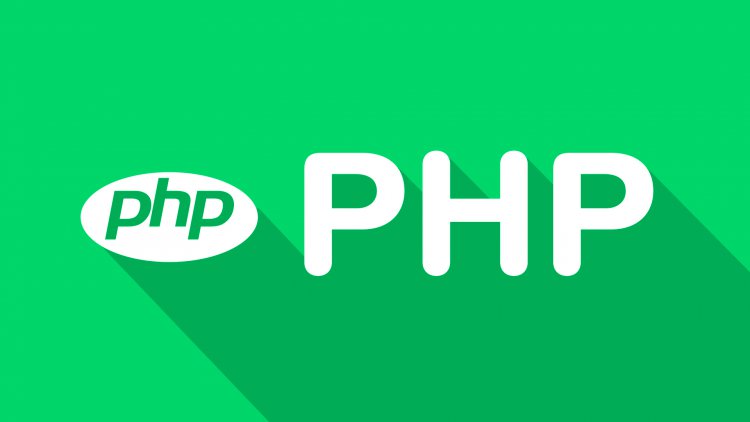
Whenever we need to integrate third-party financial API, they allow sending and receiving data in encrypted form instead of plain text.
Generally, all they need AES encryption for data security.
In this tutorial, I am going to show you how to use AES encryption and decryption in PHP.
Encryption works by taking plain text and converting it into cipher text using some methods and algorithms.
What is AES Encryption and How it Works?
AES stands for Advanced Encryption Standard. AES encryption has three block ciphers which are AES-128 (128 bit), AES-192 (192 bit), AES-256 (256 bit).
These block ciphers are named due to the key used for the encryption and decryption process.
We can encrypt our text with AES encryption and choose a key length as per requirement(128, 192, and 256 bit).
So, let us see how to use AES encryption and decryption in PHP.
AES Encryption in PHP
<?php
//Define cipher
$cipher = "aes-256-cbc";
//Generate a 256-bit encryption key
$encryption_key = openssl_random_pseudo_bytes(32);
// Generate an initialization vector
$iv_size = openssl_cipher_iv_length($cipher);
$iv = openssl_random_pseudo_bytes($iv_size);
//Data to encrypt
$data = "Sample Text";
$encrypted_data = openssl_encrypt($data, $cipher, $encryption_key, 0, $iv);
echo "Encrypted Text: " . $encrypted_data;
?>
AES Decryption in PHP
<?php //Define cipher $cipher = "aes-256-cbc"; //Decrypt data $decrypted_data = openssl_decrypt($encrypted_data, $cipher, $encryption_key, 0, $iv); echo "Decrypted Text: " . $decrypted_data; ?>
Note: If you will try to print $iv or $iv_size variable then it will show you unknown characters by default. So to see its value in readable form, you have to use the bin2hex() function.
Another important thing you have to keep in mind that decryption always required the same encryption key and initialization vector(iv) which was used for encryption.
Conclusion
In this tutorial, you have learned how to encrypt and decrypt data using AES.
If you have any queries, feel free to comment below.