How to make API secure with Hybrid encryption & decryption?
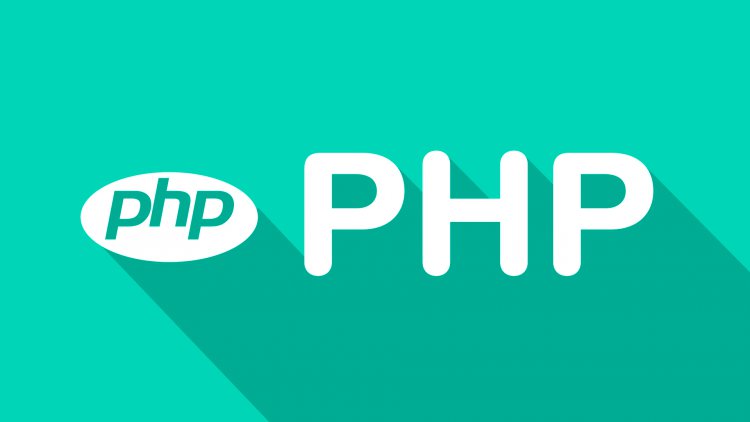
Hybrid encryption is an method to encoding and decoding data that blends the speed and convenience of a public asymmetric encryption scheme with the effectiveness of a private symmetric encryption scheme.
In this method to cryptography, the sender generates a private key (PEM), encrypts the key by using a public key algorithm and then encrypts the entire message (including the already-encrypted private key) with the original symmetric key. The encoded cipher can only be decoded if the recipient knows the private key the sender originally generated.
If User 1 wants to send an encypted message to User 2 in a hybrid cryptosystem, for example, he might do the following:
- Request User 2's public key.
- Generate a new symmetric (private) key and use it to encrypt a message.
- Use User 2's public key to encrypt the new symmetric (private) key and the message.
- Send the entire cipher to User 2'.
User 2 will then be able to use her own private key to decrypt the sender's private key and decode the rest of the message.
Security researchers are looking at ways hybrid encryption can be used as an alternative in quantum computing to more traditional encryption schemes. Until standards have been put in place, however, a hybrid approach can be accompanied by an increased risk of implementation flaws that can negate the encryption scheme's usefulness.
AES:
AES is a variant of the Rijndael block cipher developed by two Belgian cryptographers, Joan Daemen and Vincent Rijmen, who submitted a proposal to NIST during the AES selection process. Rijndael is a family of ciphers with different key and block sizes. For AES, NIST selected three members of the Rijndael family, each with a block size of 128 bits, but three different key lengths: 128, 192 and 256 bits.
Generate rsa key pair (private key/public key) with openssl:
RSA:
RSA (Rivest–Shamir–Adleman) is a public-key cryptosystem that is widely used for secure data transmission. It is also one of the oldest. The acronym "RSA" comes from the surnames of Ron Rivest, Adi Shamir and Leonard Adleman, who publicly described the algorithm in 1977. An equivalent system was developed secretly in 1973 at GCHQ (the British signals intelligence agency) by the English mathematician Clifford Cocks. That system was declassified in 1997.
Advantage and Disadvantage:
The combination of encryption methods has various advantages. One is that a connection channel is established between two users' sets of equipment. Users then have the ability to communicate through hybrid encryption.
Asymmetric encryption can slow down the encryption process, but with the simultaneous use of symmetric encryption, both forms of encryption are enhanced. The result is the added security of the transmittal process along with overall improved system performance.