Face Recognition System for Surveillance and Security Applications using Python
Discover the power of a face recognition system developed using Python for surveillance and security applications. Unlock advanced facial analysis, real-time identification, and robust security measures to protect your premises. Explore the potential of cutting-edge technology in safeguarding your environment.
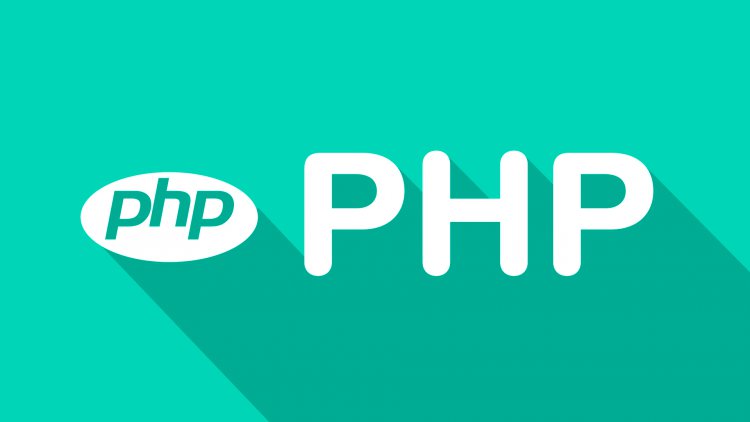
In today's security landscape, traditional surveillance systems are being complemented by advanced face recognition technology. With a Python-based face recognition system, businesses and organizations can enhance their surveillance capabilities, enabling real-time identification, proactive security measures, and effective access control. In this post, we delve into the benefits and applications of a face recognition system tailored for surveillance and security scenarios.
Unlocking Advanced Facial Analysis: Harnessing the power of Python, a face recognition system empowers security teams to perform advanced facial analysis. From detecting faces in real-time video feeds to extracting facial features and expressions, this technology provides a deeper understanding of individuals under surveillance. Python's versatile libraries such as OpenCV and dlib facilitate the implementation of robust facial analysis algorithms, enabling enhanced threat assessment and situational awareness.
Real-Time Identification for Effective Monitoring: A key advantage of a Python-based face recognition system is its ability to perform real-time identification. By comparing live video feeds against a database of known individuals, security personnel can instantly identify persons of interest or unauthorized individuals. This capability allows for proactive response, rapid threat mitigation, and improved incident management. Python's computational efficiency and machine learning frameworks like TensorFlow contribute to the system's real-time identification capabilities.
Enhanced Access Control and Intrusion Prevention: Integrating face recognition technology into access control systems provides an additional layer of security. Python's flexibility enables seamless integration with existing security infrastructure, such as surveillance cameras, door locks, and alarm systems. By authenticating individuals based on facial recognition, unauthorized access attempts can be thwarted effectively, minimizing security breaches and safeguarding sensitive areas. Python's ease of integration and compatibility with hardware interfaces like Raspberry Pi make it an ideal choice for building robust access control systems.
Scalability and Adaptability for Various Environments: Python's versatility makes it suitable for face recognition systems in diverse surveillance and security environments. Whether it's securing corporate offices, public spaces, airports, or critical infrastructure, Python-based systems can be tailored to specific requirements. The scalability and adaptability of Python allow for easy expansion and integration with evolving security technologies, ensuring long-term viability and future-proofing your surveillance infrastructure.
Addressing Privacy and Ethical Considerations: While deploying face recognition systems, privacy and ethical concerns must be carefully addressed. Organizations should establish clear policies regarding data handling, consent, and transparency. By adhering to industry best practices and regulations, including anonymizing data and implementing secure storage measures, the system can maintain a balance between security and individual privacy.
Python :
import cv2
import face_recognition
# Load the known faces and their labels
known_faces = []
known_labels = []
# Populate the known_faces and known_labels lists with pre-identified faces and corresponding labels
# Load the video feed from the surveillance camera
video_capture = cv2.VideoCapture(0)
while True:
# Capture each frame from the video feed
ret, frame = video_capture.read()
# Convert the frame to grayscale for face detection
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# Perform face detection
face_locations = face_recognition.face_locations(gray)
face_encodings = face_recognition.face_encodings(gray, face_locations)
# Loop through each detected face
for face_encoding, face_location in zip(face_encodings, face_locations):
# Compare the face encoding with the known faces
matches = face_recognition.compare_faces(known_faces, face_encoding)
# Check if there is a match
if True in matches:
matched_index = matches.index(True)
label = known_labels[matched_index]
# Draw a rectangle around the face and display the label
top, right, bottom, left = face_location
cv2.rectangle(frame, (left, top), (right, bottom), (0, 255, 0), 2)
cv2.putText(frame, label, (left, top - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.9, (0, 255, 0), 2)
# Display the resulting frame
cv2.imshow('Video', frame)
# Exit the loop if 'q' is pressed
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# Release the video capture and close the windows
video_capture.release()
cv2.destroyAllWindows()
Explanation:
- The code begins by importing the necessary libraries:
cv2
for video capture and image processing, andface_recognition
for face detection and recognition tasks. - The known faces and their corresponding labels are stored in two lists:
known_faces
andknown_labels
. These lists should be populated with pre-identified faces and their labels. - The code initializes the video capture from the surveillance camera using
cv2.VideoCapture(0)
. Adjust the parameter if you have multiple cameras or if the video feed comes from a different source. - Inside the
while
loop, each frame is captured from the video feed usingvideo_capture.read()
. - The frame is converted to grayscale using
cv2.cvtColor
to improve face detection accuracy. - Face detection is performed using
face_recognition.face_locations
. This returns a list of face bounding box coordinates. - Face encodings are extracted using
face_recognition.face_encodings
based on the detected face locations. - The face encodings are compared with the known faces using
face_recognition.compare_faces
. This returns a list of boolean values indicating whether there is a match or not. - If there is a match, the corresponding label is retrieved from the
known_labels
list. - A rectangle is drawn around the detected face using
cv2.rectangle
, and the label is displayed usingcv2.putText
. - The resulting frame with the visualizations is displayed using
cv2.imshow
. - The loop continues until the user presses 'q', at which point the loop is exited.
- Finally, the video capture is released using
video_capture.release()
and the windows are closed usingcv2.destroyAllWindows()
.
Conclusion: Python-based face recognition systems offer a powerful solution for surveillance and security applications. Leveraging advanced facial analysis, real-time identification, and enhanced access control, businesses and organizations can strengthen their security measures and protect their premises effectively. Embrace the potential of a Python-based face recognition system to create a safer and more secure environment for all.
Elevate your surveillance and security capabilities with a Python-based face recognition system. Empower your team, deter threats, and ensure peace of mind. Discover the future of surveillance technology today!