Face recognition- Face Matching Made Easy: Python-Based Face Recognition Techniques
Face recognition, Face recognition python ,Face recognition system, Facial recognition system, face matching
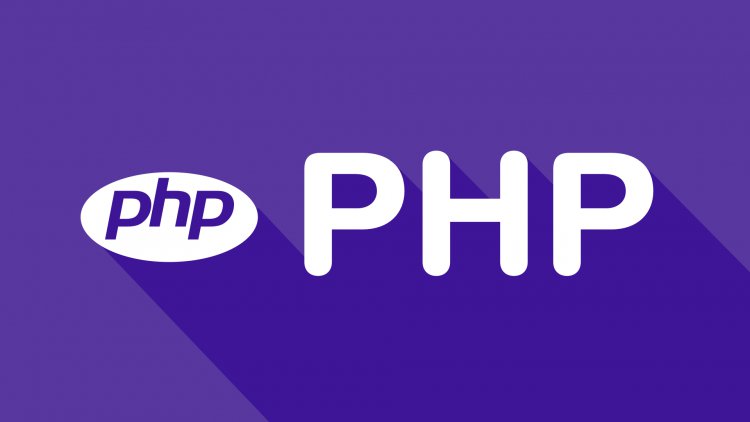
In the modern era of advanced technology, face recognition has become an integral part of various applications, ranging from security systems to personalized user experiences. With the power of Python programming, implementing face matching and recognition has become more accessible than ever before. In this post, we will dive into the realm of face matching, exploring the ease and effectiveness of Python-based techniques for accurate identification.
-
Understanding Face Recognition: To embark on the journey of face matching, it's crucial to grasp the fundamentals of face recognition. We will explore the concepts behind facial landmarks detection, face encodings, and feature extraction. By leveraging Python libraries such as OpenCV and face_recognition, we can effectively process facial data and extract meaningful information.
-
Preprocessing and Data Preparation: Before initiating the face matching process, proper preprocessing and data preparation are essential. We will discuss techniques for image cleaning, alignment, and normalization. With Python's rich ecosystem of image processing libraries, we can efficiently handle various challenges, such as varying lighting conditions and facial expressions.
-
Implementing Face Matching Algorithms: Python provides a wide range of powerful libraries and frameworks for implementing face matching algorithms. We will explore popular approaches such as Euclidean distance and deep learning-based techniques. Leveraging libraries like scikit-learn and TensorFlow, we can effortlessly compare facial features and measure similarity between faces.
-
Building an Interactive Face Matching Application: Putting our knowledge into practice, we will develop a user-friendly face matching application using Python. Through an intuitive graphical user interface (GUI), users can upload images, perform face matching operations, and receive accurate identification results in real-time. This hands-on implementation will showcase the simplicity and effectiveness of Python-based face recognition techniques.
-
Ensuring Security and Privacy: In the realm of face matching, security and privacy are of utmost importance. We will discuss techniques to enhance the security of face recognition systems, including methods for face template protection and secure data storage. Additionally, we will explore privacy considerations, ensuring that users' personal information remains protected throughout the face matching process.
Python Code:
import cv2
import face_recognition
# Load the image containing faces
image = cv2.imread('image.jpg')
# Convert the image from BGR (default format) to RGB
rgb_image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
# Detect faces in the image
face_locations = face_recognition.face_locations(rgb_image)
# Encode the faces
face_encodings = face_recognition.face_encodings(rgb_image, face_locations)
# Load a reference image for comparison (known face)
known_image = face_recognition.load_image_file('known_face.jpg')
known_face_encoding = face_recognition.face_encodings(known_image)[0]
# Iterate through the detected faces
for (top, right, bottom, left), face_encoding in zip(face_locations, face_encodings):
# Compare the detected face with the known face
matches = face_recognition.compare_faces([known_face_encoding], face_encoding)
# Calculate the Euclidean distance between the faces
face_distance = face_recognition.face_distance([known_face_encoding], face_encoding)
# Find the index of the closest match
best_match_index = face_distance.argmin()
# Check if the face matches the known face
if matches[best_match_index]:
name = "Known Person"
else:
name = "Unknown Person"
# Draw a rectangle around the face and display the name
cv2.rectangle(image, (left, top), (right, bottom), (0, 255, 0), 2)
cv2.putText(image, name, (left, top - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.9, (0, 255, 0), 2)
# Display the output image
cv2.imshow('Face Recognition', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
#Face recognition, #Face recognition python ,#Face recognition system, #Facial recognition system, #Face matching
Explanation:
- The code begins by importing the necessary libraries, namely
cv2
(OpenCV) andface_recognition
. - The image containing faces is loaded using
cv2.imread
and stored in theimage
variable. - To perform face recognition, the image needs to be converted from the default BGR format to RGB using
cv2.cvtColor
. - The
face_recognition.face_locations
function is used to detect the faces in the image. It returns a list of bounding box coordinates for each detected face. - Next, the faces are encoded using
face_recognition.face_encodings
, which generates face embeddings for each detected face. - A reference image of a known face is loaded using
face_recognition.load_image_file
, and its face encoding is obtained. - The code then iterates through the detected faces, comparing each face encoding with the known face encoding using
face_recognition.compare_faces
. - The Euclidean distance between the face encodings is calculated using
face_recognition.face_distance
. - The index of the closest match is found using
argmin
. - If a match is found, the person is labeled as "Known Person"; otherwise, they are labeled as "Unknown Person".
- Finally, a rectangle is drawn around each face using
cv2.rectangle
, and the person's name is displayed usingcv2.putText
. - The output image is displayed using
cv2.imshow
, andcv2.waitKey
waits for a key press before closing the window.
Note: Make sure to replace 'image.jpg' and 'known_face.jpg' with the actual file paths of your images.
#Face recognition, #Face recognition python ,#Face recognition system, #Facial recognition system, #Face matching
Conclusion: Face matching and recognition have become increasingly important in our technologically advanced world. With Python and its vast array of libraries and tools, implementing face recognition techniques has become more accessible, even for those with limited expertise. By following this guide, readers will gain a solid understanding of Python-based face matching techniques and be empowered to develop their own face recognition applications with ease.
Remember, with the power of Python, face matching has never been easier, unlocking a myriad of possibilities for seamless identification and personalized experiences. Let's embark on this exciting journey into the world of Python-based face recognition techniques!