How to connect AWS DynamoDB with PHP?
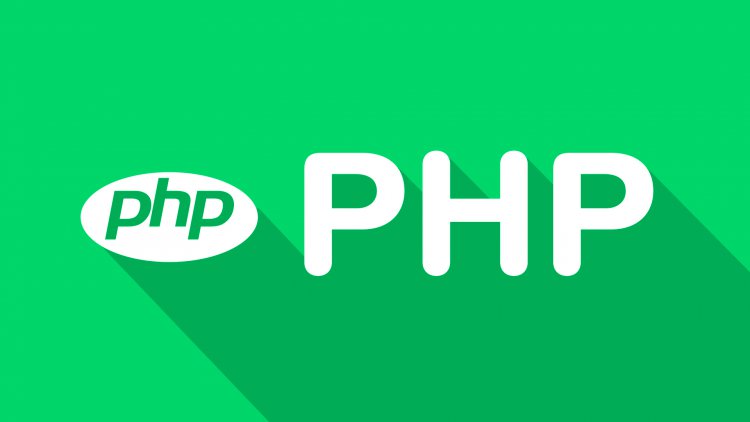
It gives you a step by step outline of the setup process for credentials and comes with a easy to use add on to the PHP SDK for AWS
AWS Setup - AWS doesn't lay out the Credentials setup for you set by step, so I will.
-
1. Go to AWS and get your PUBLIC_KEY and PRIVATE_KEY
-
2. Open Terminal
-
3. If you haven't created your credentials yet, in Terminal's fresh page, type in:
nano ~/.aws/credentials
- The
nano
function page will open up. You will seeGNU nano 2.0.6...
at the top.
- The
-
4. Inside the
nano
page, type in:[default] aws_access_key_id = public_key_ABCDEFGHIJKLMNOPQRSTUVWXYZ aws_secret_access_key = private_key_s0m3_CR42Y_l3tt3rS_i5y0ur53cr3tK3y
-
5. Once you've typed it out, hit CONTROL + X (Yes...Control, not Command).
- 6. Hit Y then ENTER
- 7. Get the [AWS_SDK_PHP][4]
- 8. Go to Your Elastic Beanstalk
- 9. When you are done creating your app and you see the Overview screen with the green check. Look to the side and hit Configuration.
- 10. Under Software Configuration -> Document root: type in: /
- 11. Under Property Name -> AWS_ACCESS_KEY_ID type in: [your_access_key]
- 12. Under AWS_ACCESS_KEY_ID is the AWS_SECRET_KEY, type in: [your_secret_key]
- 13. When your PHP Project is ready. Put all your files into one folder. Name the folder [whatever], then compress the files inside [whatever]. Do not compress the whole folder. Only compress the files in the folder. If one of these files includes your index.php or index.html, your project will show up on EBS default URL.
- 14. Your project should be called Archive.zip (Mac). Go to EBS, upload the zip and there you go! All finished with AWS Setup!
AWS Setup
- 1. Put the AWS_SDK_PHP in an empty folder
-
2. At the top of the file using the SDK (
index.php
or whatever), put in:require 'aws/aws-autoloader.php'; date_default_timezone_set('America/New_York'); use Aws\DynamoDb\DynamoDbClient; $client = new DynamoDbClient([ 'profile' => 'default', 'region' => 'us-east-1', 'version' => 'latest' ]);
- Data Types
- S = String
- N = Number
- B = Binary
-
The Basic Methods
-
Describe Table
$result = $client->describeTable(array( 'TableName' => '[Table_Name]' )); echo $result;
-
Put Item
$response = $client->putItem(array( 'TableName' => '[Table_Name]', 'Item' => array( '[Hash_Name]' => array('S' => '[Hash_Value]'), '[Range_Name]' => array('S' => '[Range_Value]') ) )); //Echoing the response is only good to check if it was successful. Status: 200 = Success echo $response;
-
Get Item
$response = $client->getItem(array( 'TableName' => '[Table_Name]', 'Key' => array( '[Hash_Name]' => array('S' => '[Hash_Value]'), '[Range_Name]' => array('S' => '[Range_Value]') ) )); echo $response;
-
Delete Item
$response = $client->deleteItem(array( 'TableName' => '[Table_Name]', 'Key' => array( '[Hash_Name]' => array('S' => '[Hash_Value]'), '[Range_Name]' => array('S' => '[Range_Value]') ) )); //Echoing the response is only good to check if it was successful. Status: 200 = Success echo $response;
-
Query Item
$response = $client->query(array( 'TableName' => '[Table_Name]', 'KeyConditionExpression' => '[Hash_Name] = :v_hash and [Range_Name] = :v_range', 'ExpressionAttributeValues' => array ( ':v_hash' => array('S' => '[Hash_Value]'), ':v_range' => array('S' => '[Range_Value]') ) )); echo $response;
-
Hope this helps.