Image Conversion and Cleaning Script using Python Imaging Library
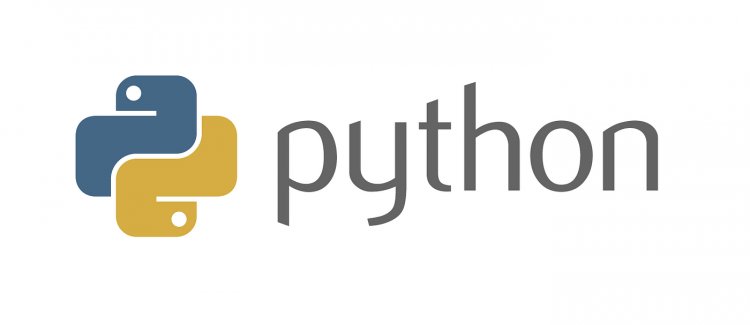
In the world of digital media, image files often require conversion and cleaning for various purposes. Python developers can leverage the power of the Python Imaging Library (PIL) to automate these tasks efficiently. This article explores a Python script that utilizes PIL to convert and clean image files, offering a streamlined solution for image processing.
Script Overview:
The Python script presented here consists of two key functions: web2jpg and cleaner. Let's take a closer look at each function's functionality and how they contribute to the overall image conversion and cleaning process.
The web2jpg Function:
The web2jpg function is designed to convert image files to the JPEG format. It accepts a filename as input and utilizes PIL to open the image. The image is then converted to RGB format to ensure compatibility with JPEG encoding. Finally, the function saves the converted image as a new JPEG file, using the same base name as the original image.
The cleaner Function:
The cleaner function focuses on cleaning image files by removing Exif metadata. Similar to the web2jpg function, it takes a filename as input and opens the image using PIL. The function extracts the image data, creates a new image with the same mode and size, and puts the data into the new image, effectively removing the Exif metadata. The cleaned image is then saved with a filename prefixed with "clean_".
Script Execution:
After defining the two functions, the script proceeds to iterate through the files in the current directory. It identifies image files with specific extensions, such as .jpeg, .jpg, and .webp. For each identified image file, the script applies the appropriate conversion and cleaning operations using the web2jpg and cleaner functions.
Error Handling: The script includes error handling to account for potential issues during execution. If an unknown file format is encountered, the script prints a message indicating that the format is not supported. Additionally, any exceptions that occur during the execution of the script are caught, and an appropriate error message is displayed to the user.
Code:
# Uses the Python Imaging Library
# Code & Compiled By: dharambir Singh
# `pip install Pillow` works too
import os
from PIL import Image
import pillow_avif
def web2jpg(filename):
im = Image.open(filename).convert("RGB")
fn = os.path.splitext(os.path.basename(filename))[0]
return im.save(fn+".jpg","jpeg")
def cleaner(filename):
image = Image.open(filename)
# next 3 lines strip exif
image_data = list(image.getdata())
image_without_exif = Image.new(image.mode, image.size)
image_without_exif.putdata(image_data)
return image_without_exif.save(u"clean_{}".format(filename), quality=95, optimize=True)
def avif2jpg(filename):
img = Image.open(filename)
fn = os.path.splitext(os.path.basename(filename))[0]
return img.save(fn+".jpg","jpeg")
def removeExistingFile(filename):
return os.remove(filename)
try:
for x in os.listdir():
if x.endswith(".jpeg") or x.endswith(".jpg") or x.endswith(".png"):
# Prints only text file present in My Folder
print(x)
cleaner(x)
removeExistingFile(x)
elif x.endswith(".webp"):
print(x)
fn1 = web2jpg(x)
cleaner(fn1)
removeExistingFile(x)
elif x.endswith(".avif"):
print(x)
fn1 = avif2jpg(x)
cleaner(fn1)
removeExistingFile(x)
else:
print("Unknown format for us.")
except:
print("An exception occurred")
Explanation:
This Python script utilizes the Python Imaging Library (PIL) to convert and clean image files. The script includes two functions: web2jpg and cleaner.
The web2jpg function takes a filename as input, opens the image using PIL, converts it to RGB format, and saves it as a JPEG file with the same base name as the original image.
The cleaner function opens an image file, removes the Exif metadata from the image, and saves the cleaned image with a filename prefixed with "clean_".
The script then iterates through the files in the current directory, identifies the image files with specific extensions (.jpeg, .jpg, .webp), and performs the corresponding conversion and cleaning operations using the defined functions.
If an unknown file format is encountered, a message is printed indicating that the format is not supported. If any exception occurs during the execution of the script, an appropriate error message is displayed.
Conclusion:
Automating image conversion and cleaning tasks can greatly simplify workflows when dealing with a large number of image files. The Python script showcased in this article, powered by the Python Imaging Library (PIL), offers a convenient solution for converting images to the JPEG format and removing Exif metadata. By leveraging this script, developers can save time and effort while efficiently processing image files for their specific needs.