MongoDB - Advanced Indexing
MongoDB - Advanced Indexing
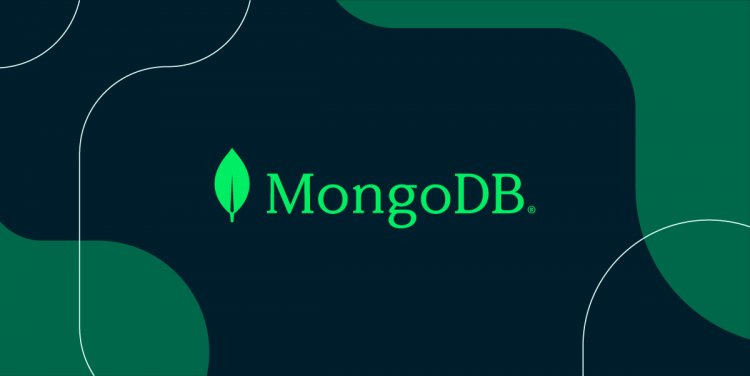
MongoDB - Advanced Indexing
In the ever-evolving landscape of database management, MongoDB stands tall as a dynamic NoSQL database. One of its standout features is indexing, a powerful tool that can significantly enhance your database's performance. In this article, we dive into advanced indexing techniques in MongoDB, backed by real-world examples. Whether you're a seasoned developer or just stepping into the world of MongoDB, you'll find valuable insights to optimize your database queries.
Table of Contents
- Introduction to MongoDB Indexing
- The Power of Basic Indexes
- Crafting Compound Indexes
- Text Indexes for Full-Text Search
- GeoSpatial Indexing for Location-Based Data
- Indexing Arrays and Subdocuments
- Wildcard Indexing with Wildcard Text Search
- Strategies for Indexing Large Data Sets
- Real-World Examples
- Conclusion
- FAQs
- Interview Question and Answer
1. Introduction to MongoDB Indexing
Before we delve into advanced indexing, let's recap the basics. An index is like the index of a book, enabling you to find information quickly within a collection. MongoDB uses B-tree indexes, making data retrieval efficient.
2. The Power of Basic Indexes
Indexes are a powerful tool in MongoDB that can be used to improve the performance of queries. They do this by creating a copy of the data in a specific field or set of fields, and then ordering the data in a way that makes it easy for MongoDB to find the relevant documents.
There are two main types of indexes in MongoDB:
- Single-field indexes are indexes on a single field. They are the most common type of index, and they are used to improve the performance of queries that filter on a single field.
- Compound indexes are indexes on multiple fields. They are used to improve the performance of queries that filter on multiple fields.
Indexes can be created on any field in a MongoDB collection. However, not all fields are created equal. The most effective indexes are those that are used frequently in queries.
3. Crafting Compound Indexes
Compound indexes in MongoDB are indexes that are created on multiple fields. They are used to improve the performance of queries that filter on multiple fields.
To create a compound index, you need to specify the names of the fields in the order that you want them to be sorted. For example, the following command creates a compound index on the item
and quantity
fields:
db.collection.createIndex({item: 1, quantity: 1});
The 1
after each field name indicates that the field should be sorted in ascending order. You can also specify -1
to sort the field in descending order.
Compound indexes can be used to improve the performance of queries that filter on any combination of the fields in the index. For example, the following query will use the compound index to find all documents where the item
field is equal to "apple" and the quantity
field is greater than 10:
db.collection.find({item: "apple", quantity: {gt: 10}});
Compound indexes can significantly improve the performance of queries that filter on multiple fields. However, they also take up more storage space than single-field indexes. Therefore, it is important to only create compound indexes on fields that are used frequently in queries.
4. Text Indexes for Full-Text Search
Text indexes in MongoDB are used to improve the performance of full-text search queries. They do this by creating a copy of the data in the text field, and then indexing the data in a way that makes it easy for MongoDB to find the documents that contain the search terms.
To create a text index, you need to use the createIndex()
method and specify the text
option. For example, the following command creates a text index on the title
field:
db.collection.createIndex({title: "text"});
The text
option tells MongoDB to create a text index on the title
field.
Text indexes can be used to improve the performance of a variety of full-text search queries, such as:
- Finding documents that contain a specific word or phrase.
- Finding documents that contain all of the words in a phrase.
- Finding documents that contain words that are related to each other.
Text indexes can significantly improve the performance of full-text search queries. However, they also take up more storage space than other types of indexes. Therefore, it is important to only create text indexes on fields that are used frequently in full-text search queries.
5. GeoSpatial Indexing for Location-Based Data
Geospatial indexing in MongoDB is a way to improve the performance of queries that involve location data. MongoDB supports two types of geospatial indexes:
- 2d indexes: These indexes are used for data that is stored as legacy coordinate pairs.
- 2dsphere indexes: These indexes are used for data that is stored as GeoJSON objects.
Geospatial indexes are created on fields that contain location data. For example, if you have a collection of documents that store the location of a restaurant, you could create a geospatial index on the location
field.
Geospatial indexes can be used to improve the performance of a variety of queries, such as:
- Finding all restaurants within a certain radius of a given point.
- Finding all restaurants that are located in a certain area.
- Finding all restaurants that are on a certain street.
Geospatial indexes can significantly improve the performance of these queries. However, they also take up more storage space than other types of indexes. Therefore, it is important to only create geospatial indexes on fields that are used frequently in geospatial queries.
6. Indexing Arrays and Subdocuments
Yes, it is possible to index arrays and subdocuments in MongoDB.
- Indexing arrays: You can index an array by specifying the name of the array field in the
createIndex()
method. For example, the following command creates an index on theitems
array field:
db.collection.createIndex({items: 1});
The 1
after the items
field name indicates that the array should be sorted in ascending order. You can also specify -1
to sort the array in descending order.
- Indexing subdocuments: You can index a subdocument by specifying the name of the subdocument field in the
createIndex()
method. For example, the following command creates an index on theuser
subdocument field:
db.collection.createIndex({user: {name: 1}});
The name
field inside the user
subdocument will be sorted in ascending order.
Indexes on arrays and subdocuments can be used to improve the performance of queries that filter on the values in the array or subdocument. For example, the following query will use the index on the items
array field to find all documents where the items
array contains the value "apple":
db.collection.find({items: "apple"});
Indexes on arrays and subdocuments can significantly improve the performance of these queries. However, they also take up more storage space than indexes on simple fields. Therefore, it is important to only create indexes on arrays and subdocuments that are used frequently in queries.
7. Wildcard Indexing with Wildcard Text Search
Wildcard indexing is a type of indexing that allows you to index all documents in a collection that have a field with a value that matches a wildcard pattern. For example, if you have a collection of documents that store the name of a product, you could create a wildcard index on the name
field. This would allow you to perform wildcard text searches on the name
field, such as finding all documents that have a name that starts with the letter "a".
Wildcard text search is a type of text search that allows you to search for documents that contain a word or phrase that matches a wildcard pattern. For example, if you have a collection of documents that store the description of a product, you could perform a wildcard text search on the description
field to find all documents that contain the word "apple".
To use wildcard indexing with wildcard text search, you need to create a wildcard index on the field that you want to search. You can then use the $text
operator in your queries to perform wildcard text searches.
The following example shows how to create a wildcard index on the name
field and then perform a wildcard text search on the name
field:
db.collection.createIndex({name: "*"});
db.collection.find({name: { $text: { $search: "a*" } }});
The first line creates a wildcard index on the name
field. The second line performs a wildcard text search on the name
field. The $text
operator specifies that the query is a text search. The $search
operator specifies the wildcard pattern that you want to match. In this case, the wildcard pattern is "a*". This pattern matches any word that starts with the letter "a".
Wildcard indexing with wildcard text search can be a powerful way to search for documents in a MongoDB collection. However, it is important to note that wildcard indexing can make your collection larger and slower. Therefore, it is important to only create wildcard indexes on fields that are used frequently in wildcard text searches.
8. Strategies for Indexing Large Data Sets
Large data sets require careful indexing strategies. We discuss techniques for managing and optimizing indexes in high-volume scenarios.
here are some strategies for indexing large data sets in MongoDB:
- Use compound indexes: Compound indexes are indexes on multiple fields. They can be used to improve the performance of queries that filter on multiple fields.
- Use text indexes: Text indexes are indexes on text fields. They can be used to improve the performance of full-text search queries.
- Use geospatial indexes: Geospatial indexes are indexes on location fields. They can be used to improve the performance of queries that involve location data.
- Use sparse indexes: Sparse indexes are indexes on fields that are not always populated. They can save storage space and improve write performance.
- Use partial indexes: Partial indexes are indexes on a subset of the values in a field. They can be used to improve the performance of queries that filter on a specific range of values.
- Use materialized views: Materialized views are pre-computed views of the data. They can be used to improve the performance of queries that are frequently executed.
- Shard your data: Sharding is a technique for dividing a large data set across multiple servers. This can improve the performance of queries by distributing the load across multiple servers.
9. Real-World Examples
We bring theory to life with real-world examples. From e-commerce product catalogs to geolocation-based services, we demonstrate how advanced indexing can solve complex challenges.
Here are some real-world examples of how indexes are used in MongoDB:
- Ecommerce: An ecommerce website could use indexes on the product name, product category, and product price fields to improve the performance of queries that search for products or filter products by category or price.
- Social media: A social media platform could use indexes on the user name, user location, and post content fields to improve the performance of queries that search for users, filter posts by location, or find posts that contain a specific keyword.
- Log analysis: A log analysis system could use indexes on the log timestamp, log source, and log message fields to improve the performance of queries that search for logs or filter logs by source or message.
- Fraud detection: A fraud detection system could use indexes on the transaction amount, transaction time, and customer IP address fields to improve the performance of queries that identify fraudulent transactions.
- Real-time analytics: A real-time analytics system could use indexes on the event timestamp, event type, and event value fields to improve the performance of queries that analyze event data in real time.
10. Conclusion
As we conclude this journey into MongoDB's advanced indexing techniques, you'll be equipped to leverage indexing for optimal database performance. Whether it's improving text search, handling geospatial data, or managing large datasets, MongoDB's indexing capabilities can be a game-changer for your applications.
FAQs
1. What is the primary purpose of indexing in MongoDB?
- Indexing in MongoDB is primarily used to improve query performance by enabling efficient data retrieval.
2. Are compound indexes always more efficient than single-field indexes?
- No, compound indexes are efficient for specific queries but may not be the best choice for all scenarios. The choice depends on query patterns.
3. How can I ensure that my MongoDB indexes remain optimized over time?
- Regularly monitor your database's query performance and use MongoDB's built-in tools for index analysis and optimization.
4. Can I create custom indexes for complex data types like arrays or subdocuments?
- Yes, MongoDB allows you to create custom indexes tailored to your data's structure and query requirements.
5. Are there any limitations to the number of indexes I can create in MongoDB?
- Yes, MongoDB has limits on the number and size of indexes per collection. It's essential to plan your indexes carefully to stay within these limits.
12. Interview Question and Answer
Question: What is a compound index in MongoDB?
Answer: A compound index is an index on multiple fields. It can be used to improve the performance of queries that filter on multiple fields. For example, a compound index on the product_name
and product_category
fields could be used to improve the performance of a query that searches for products by name and category.
Question: What is a partial index in MongoDB?
Answer: A partial index is an index on a subset of the values in a field. It can be used to improve the performance of queries that filter on a specific range of values. For example, a partial index on the product_price
field could be used to improve the performance of a query that finds products with prices between $100 and $200.
Question: What is a sparse index in MongoDB?
Answer: A sparse index is an index on fields that are not always populated. It can save storage space and improve write performance. For example, a sparse index on the email
field could be used to improve the performance of queries that search for documents by email address, but it would not be used to index documents that do not have an email address.
Question: What are the different types of geospatial indexes in MongoDB?
Answer: MongoDB supports two types of geospatial indexes:
- 2d indexes: These indexes are used for data that is stored as legacy coordinate pairs.
- 2dsphere indexes: These indexes are used for data that is stored as GeoJSON objects.
Question: What are the benefits of using indexes in MongoDB?
Answer: Indexes can improve the performance of queries in a number of ways:
- They can reduce the number of documents that need to be scanned to find the relevant documents.
- They can improve the order in which documents are returned, which can make it easier to find the relevant documents.
- They can reduce the amount of data that needs to be read from disk, which can improve performance.
Question: What are the drawbacks of using indexes in MongoDB?
Answer: Indexes can have a few drawbacks:
- They can take up additional storage space.
- They can slow down write operations.
- They need to be updated whenever a document is inserted, updated, or deleted, which can add overhead.
Question: How can I choose the right indexes for my MongoDB collection?
Answer: The best way to choose the right indexes for your MongoDB collection is to consider the following factors:
- The queries that you need to perform.
- The fields that are used frequently in those queries.
- The size of your collection.
- The amount of storage space that you have available.
- The performance requirements of your application.
By carefully considering these factors, you can choose the indexes that will give you the best performance for your specific needs.