Face Recognition-based Smart Door Lock System using Python and Arduino
Discover how to enhance home security with a cutting-edge smart door lock system that incorporates face recognition technology, powered by Python and Arduino. Learn how this innovative solution provides convenient and secure access control, leveraging the power of face recognition for a seamless user experience.
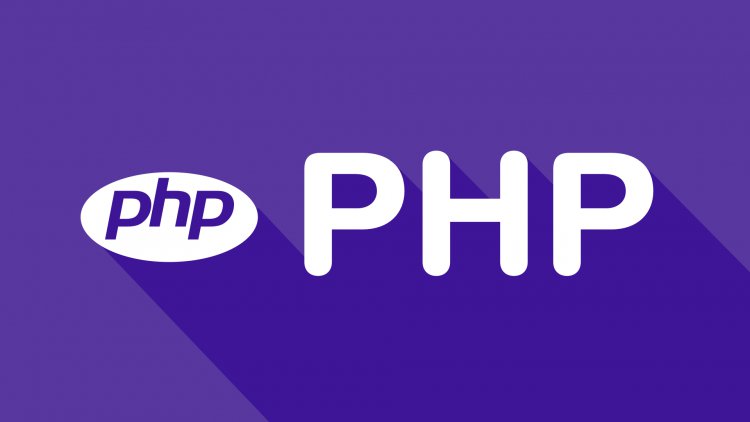
Upgrade your home security with a state-of-the-art smart door lock system that combines the advanced capabilities of face recognition technology, Python programming, and Arduino hardware. This comprehensive guide explores how to develop a secure and convenient access control system that ensures only authorized individuals can enter your premises.
Securing Your Home with Face Recognition: Face recognition technology has revolutionized security systems by offering accurate and reliable identification capabilities. By harnessing the power of Python and Arduino, we can develop a sophisticated smart lock system that recognizes authorized individuals based on their facial features. Say goodbye to traditional key-based locks and embrace the convenience and enhanced security of face recognition.
Building a Smart Door Lock System: In this tutorial, we will walk you through the step-by-step process of creating a face recognition-based smart door lock system. By combining Python's face recognition libraries and Arduino's hardware components, we will enable seamless integration between the two technologies. You will learn how to capture and process facial data, implement face recognition algorithms, and control the door lock mechanism using Arduino.
Python-Powered Face Recognition: Leveraging Python's extensive libraries such as OpenCV and face_recognition, we will explore facial feature extraction, face encoding, and matching techniques. You will discover how to train the system with authorized users' facial data, allowing it to accurately recognize and authenticate individuals in real-time. Python's versatility and simplicity make it an ideal choice for implementing robust face recognition algorithms.
Arduino-Enabled Door Lock Mechanism: Complementing the face recognition capabilities, we will utilize Arduino's hardware platform to control the door lock mechanism. By connecting Arduino components such as servo motors or solenoids, you will learn how to create a secure and responsive locking system. Arduino's compatibility with Python allows seamless communication between the face recognition module and the door lock, ensuring a synchronized and efficient operation.
Enhanced Security and Convenience: The face recognition-based smart door lock system not only enhances security but also offers unmatched convenience. With a registered face, authorized users can effortlessly access their homes without the need for keys or passcodes. The system eliminates the risk of lost or stolen keys and provides a seamless user experience, making it an ideal choice for modern homeowners.
Python Code:
import cv2
import face_recognition
import serial
# Initialize Arduino connection
arduino = serial.Serial('COM3', 9600) # Replace 'COM3' with the appropriate port
# Load the known face image and encode it
known_image = face_recognition.load_image_file('known_face.jpg')
known_face_encoding = face_recognition.face_encodings(known_image)[0]
# Initialize the webcam
video_capture = cv2.VideoCapture(0)
while True:
# Capture frame-by-frame from the webcam
ret, frame = video_capture.read()
# Convert the frame from BGR to RGB
rgb_frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
# Find all the faces in the frame
face_locations = face_recognition.face_locations(rgb_frame)
face_encodings = face_recognition.face_encodings(rgb_frame, face_locations)
for face_encoding in face_encodings:
# Compare the face with the known face
matches = face_recognition.compare_faces([known_face_encoding], face_encoding)
if True in matches:
# Face recognized, unlock the door
arduino.write(b'1') # Send '1' to Arduino
else:
# Face not recognized, lock the door
arduino.write(b'0') # Send '0' to Arduino
# Display the resulting frame
cv2.imshow('Smart Door Lock', frame)
# Exit the loop if 'q' is pressed
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# Release the webcam and close all windows
video_capture.release()
cv2.destroyAllWindows()
Explain:
- The code begins by importing the necessary libraries:
cv2
for image processing,face_recognition
for face recognition, andserial
for communication with the Arduino. - The Arduino connection is initialized using the
serial.Serial
function, specifying the appropriate port (e.g., 'COM3') and baud rate (9600). - The known face image is loaded using
face_recognition.load_image_file
and encoded usingface_recognition.face_encodings
. - The webcam is initialized using
cv2.VideoCapture(0)
, with 0 representing the default webcam device. - Inside the main loop, the code captures frames from the webcam using
video_capture.read()
. - The captured frame is converted from BGR to RGB format using
cv2.cvtColor
. - Face locations in the frame are detected using
face_recognition.face_locations
, and face encodings are generated usingface_recognition.face_encodings
. - For each face encoding, the code compares it with the known face encoding using
face_recognition.compare_faces
. - If a match is found, the Arduino is instructed to unlock the door by sending '1' through
arduino.write(b'1')
. Otherwise, it sends '0' to lock the door. - The frame is displayed using
cv2.imshow
, and the loop continues until the 'q' key is pressed. - Finally, the webcam is released and all windows are closed using
video_capture.release()
andcv2.destroyAllWindows()
.
Arduino Code:
#include
Servo lockServo;
int unlockPosition = 0; // Replace with appropriate unlock position
int lockPosition = 90; // Replace with appropriate lock position
void setup() {
lockServo.attach(9); // Replace '9' with the appropriate servo pin
pinMode(13, OUTPUT); // Replace '13' with the appropriate LED pin
Serial.begin(9600);
}
void loop() {
if (Serial.available() > 0) {
char receivedChar = Serial.read();
if (receivedChar == '1') {
unlockDoor();
} else if (receivedChar == '0') {
lockDoor();
}
}
}
void unlockDoor() {
digitalWrite(13, HIGH); // Turn on the LED to indicate unlocking
lockServo.write(unlockPosition);
delay(2000); // Adjust the delay time according to the door mechanism
}
void lockDoor() {
digitalWrite(13, LOW); // Turn off the LED to indicate locking
lockServo.write(lockPosition);
delay(2000); // Adjust the delay time according to the door mechanism
}
Explain:
- The code starts by including the
Servo
library and defining variables for the unlock and lock positions of the servo motor. - In the
setup()
function, the servo motor is attached to a specific pin (lockServo.attach(9)
), and the LED pin for indicating the lock status is set as an output (pinMode(13, OUTPUT)
). - The
loop()
function continuously checks if there is serial data available from the Python code. - If data is available, it reads the received character and performs the corresponding action:
- If '1' is received, it calls the
unlockDoor()
function. - If '0' is received, it calls the
lockDoor()
function.
- If '1' is received, it calls the
- The
unlockDoor()
function turns on the LED to indicate unlocking, moves the servo motor to the unlock position (lockServo.write(unlockPosition)
), and introduces a delay to allow the door to unlock. - The
lockDoor()
function turns off the LED to indicate locking, moves the servo motor to the lock position (lockServo.write(lockPosition)
), and introduces a delay to allow the door to lock.
Conclusion: By integrating face recognition technology, Python programming, and Arduino hardware, you can build a cutting-edge smart door lock system that provides superior security and convenience. Experience the peace of mind that comes with knowing your home is protected by an advanced access control system. Upgrade to a face recognition-based smart lock system and take your home security to new heights.