PHP Objects and Classes
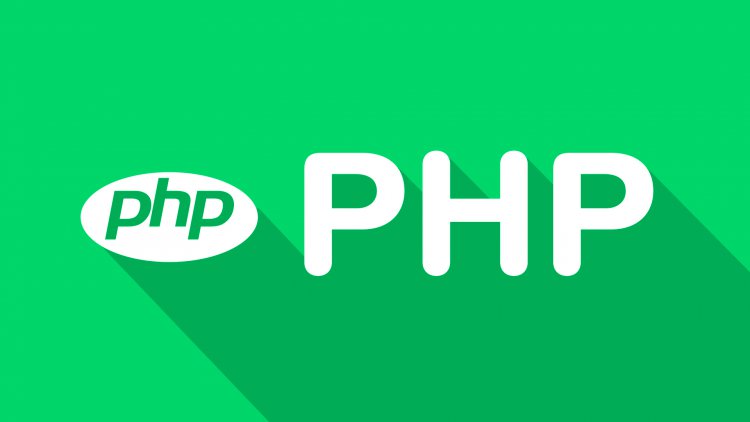
Objects
Objects in PHP are just another data type. An object allows you to store in a variable a set of properties and their values, as well as built-in functions. This makes objects similar in structure to associative arrays. But there is still a difference from arrays, and at the same time it is quite important - objects can have an internal state.
Features of objects and their differences from arrays
Let's see what a PHP object is. As stated above, objects are similar to arrays, but with their own peculiarities. Objects can contain separate values, each under its own key. These values ​​are called properties of the object.
Also, objects can have functions inside them - they are called methods of the object. Methods can access any properties of an object, read and write data there.
The value of an object property can be of any type: number, string, array, other object. But, unlike an array, objects do not allow new values ​​to be added to themselves. That is, an object always has a finite number of its properties and methods. You can change the values ​​of existing properties, but you cannot delete or replace them. Which is fundamentally different from the behavior of an array, because you can add and remove values ​​there at any time.
But the biggest thing about objects is how they are created. If the array is created either empty or immediately with a set of values, then the objects are arranged differently. The point is that objects do not exist on their own. To create a new object, you first have to create its description - a class.
A class is like a blueprint of an object. A class describes what an object is made of. We'll deal with classes a bit later.
Anatomy of an object
How does the object work from the inside? Its contents can be divided into two groups: properties and methods.
Properties can be of two types: public and hidden. Public properties can be accessed outside of the object, just like you access array elements using their keys.
Hidden properties have no analogues in the array. They are available for reading and changing only inside the object itself - and this can be done by its methods.
The second group is the methods of the object.
The collection of methods is also called object behavior. Like properties, methods are public and hidden. Public methods of an object can be called from external code, and hidden methods only from the object itself. Methods are capable of accessing object properties as easily as if they were their internal variables or arguments.
Classes
A class is a template by which objects are created.
Let me remind you that classes are descriptions of objects. We cannot create an object "on the fly", as is the case with arrays. An object can only be created based on its description - a class. This, by the way, differs from the implementation of objects in PHP from JavaScript. In JS, objects do not need any classes, and they can be created and modified whenever and wherever you want.
Class as drawing
Why are classes needed, and why objects cannot exist without them?
The analogy here is very simple: a class is a drawing, the most detailed description of how a product should look like. The class itself is not something physical and tangible, that is, we cannot use it directly in the code. Instead, a class is a schema, a structure from which the object will be created.
Object life cycle
Any work with objects in PHP consists of the following steps.
It all starts with creating a class. In the class, we fix what properties and methods each of its instances will consist of. Also in the class, you can set initial values ​​for each property.
Having a class, it is possible to create an instance of it - an object.
It is customary for PHP classes to be stored in separate files, so first you include this script where it is needed. Then you call the procedure to create a new object based on this class.
To use the object in the future, it should, as always, be assigned to a variable. Then you will work with the object through a variable: call methods and access properties.
An example of creating an object based on a class
Class description:
class WeatherEntry
{
private $date;
private $comment = "";
private $temperature = 0;
private $isRainy = false;
public function __construct($date, string $comment, int $temperature)
{
$this->date = $date;
$this->comment = $comment;
$this->temperature = $temperature;
}
public function isCold()
{
return $this->temperature < 0;
}
public function setRainStatus($rain_status)
{
$this->isRainy = $rain_status;
}
public function getDayDescription()
{
$dt = strtotime($this->date);
$delta = time() - $dt;
$days = ceil($delta / 86400);
$res = "That was $days days ago. That day there was";
if ($this->isCold()) {
$res .= "
cold. "; } else { $res .= "
pretty warm."; } if ($this->isRainy) { $res .= "The rain minced."; } else { $res .= "There was not a cloud in the sky.";
} return $res; } }
Creating an object based on a class:
$firstSeptember = new WeatherEntry("2018-09-01", "
Knowledge day", 14);
$firstSeptember->setRainStatus(false);
print($firstSeptember->getDayDescription());
Parsing an example
Let's figure out what's going on here.
Let's start with the goals of creating this class. Its task is to store weather data for a specific day in an object, as well as provide a summary for that day in text form.
There are four hidden properties defined in the class. This means that they will not be accessed outside the object. Only internal methods of the object can read and write these properties. The properties themselves store temperature parameters (temperature, precipitation), date and an additional comment to the record. Some properties have a default value.
Next comes the enumeration of methods. And it all starts with a method that has a special name and meaning - __construct
.
What is an object constructor
Object methods are called from external code when they are explicitly called with a name. But if you name one method, __construct
then it will be called automatically at the time of creating an object based on the class.
Object constructors are used to initialize values ​​and perform other preparatory operations. In our example, the constructor sets the content of the hidden properties.
Referring to properties and methods of an object
Let's see how the call to its properties occurs inside the method.
First, for this, a special variable this is used, which is always present inside the object and refers to it itself.
Secondly, to access methods and properties of an object, you need a special syntax: "arrow". This arrow separates the name of a property or method from the name of an object. This is analogous to square brackets when working with arrays.
The method with the name is isCold()
needed to find out if it was cold that day, based on the temperature readings in degrees.
The method setRainStatus()
sets a boolean value that shows the precipitation status on the day of observation.
The method getDayDescription()
generates a text description of the weather for a given date.
Creating an object based on a class
Having written the class, we have done most of the work. Now we have to create a new object based on this class and show how to work with it.
A new object is created using the new keyword, followed by the name of its class. In parentheses, you must pass all the arguments to the method __construct
, if it was written. The class is not required to contain this method, and if it does not exist, then the parentheses are optional.
In the code, we pass almost all the parameters of weather observations to the constructor. Then, for the created object, its methods are called: the first sets the precipitation values, and the second returns a text description of the weather.